Creating Your Own DeepPartial Type in Typescript to Allow Any Subset of Object Properties Deeply
Nick Scialli
September 26, 2020
You may know of the Partial utility type in Typescript, but it turns out we can create our own DeepPartial type through some clever recursive typing.
TL;DR
If you just want the type, here it is! If you want to read more about the problem, read on!
type DeepPartial<T> = Partial<{ [P in keyof T]: DeepPartial<T[P]> }>;
What is DeepPartial Type
Let’s say you have an object with properties that each have their own objects. If we use the Partial
type, we are only allowing a subset of the highest level object’s keys, but not the lower level object.
If we have the following type:
type LivingThings = {
animals: {
mammals: {
dogs: 'happy';
cats: 'annoyed';
};
};
plants: {
trees: 'tall';
ferns: 'short';
};
};
And we tried to make the following typing work:
const dogs: Partial<LivingThings> = {
animals: {
mammals: {
dogs: 'happy',
},
},
};
It would fail!
That’s because we’re allowed to have a partial object at the top level, but down within the animals
and mammals
keys, we have to include everything.
This is where a deep partial comes in handy!
Writing the DeepPartial Type
Our DeepPartial
type will also take a generic to specify the object. However, instead of just being a Partial
of our object, it will be a Partial
of our object whose keys are each mapped to a DeepPartial
of themselves!
See what I mean below:
type DeepPartial<T> = Partial<{ [P in keyof T]: DeepPartial<T[P]> }>;
Now, our DeepPartial
typing works!
const dogs: DeepPartial<LivingThings> = {
animals: {
mammals: {
dogs: 'happy',
},
},
};
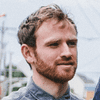
Nick Scialli is a senior UI engineer at Microsoft.