Fix the "Uncaught TypeError: destroy is not a function" Error in React
Nick Scialli
February 21, 2021
This React error seems particularly cryptic, but ends up making a lot of sense when you get the hang of the useEffect
hook.
Uncaught TypeError: destroy is not a function
It turns out this almost always happens when you try to return anything from your useEffect
hook that is not a function. For example, you might be doing something like this:
useEffect(() => callSomeAPI());
Which is the same as doing something like this:
useEffect(() => {
return callSomeAPI();
});
Why Doesn’t This Work?
If you return anything from a useEffect function, it must be a function.
The Quick Solution
The quickest fix to our issue is to, therefore, just not return anything from the effect!
useEffect(() => {
callSomeAPI();
});
And that will almost certainly work.
An Explainer: The useEffect Cleanup Function
If you return anything from the useEffect
hook function, it must be a cleanup function. This function will run when the component unmounts. This can be thought of as roughly equivalent to the componentWillUnmount
lifecycle method in class components.
The following is an example of a useEffect
that returns something it’s allowed to return (a function). Furthermore, if we were to test this in action, we’d find that the text "This will be logged on unmount"
indeed gets logged when our component unmounts.
useEffect(() => {
callSomeAPI();
return () => {
console.log('This will be logged on unmount');
};
});
And that’s all there is to it! Not the most intuitive of errors, but a quick and easy fix. Good luck!
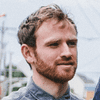
Nick Scialli is a senior UI engineer at Microsoft.