How to Abort a Fetch Request in JavaScript using AbortController
Nick Scialli
November 10, 2020
Sometimes it’s necessary to abort a fetch request. In this post, we explore how to quickly do so using AbortController!
A Simple Fetch Request
Let’s start out with a simple fetch request. We’ll grab some metadata about my Github account and log it to the console.
fetch('https://api.github.com/users/nas5w')
.then((res) => res.json())
.then((data) => {
console.log(data);
});
If we check our console, we see a json object describing my account has been logged. Here’s a bit of that data.
{"login":"nas5w","id":7538045,"node_id":"MDQ6VXNlcjc1MzgwNDU=","avatar_url":"https://avatars2.githubusercontent.com/u/7538045?v=4","gravatar_id":"","url":"https://api.github.com/users/nas5w","html_url":"https://github.com/nas5w"...}
Using AbortController
In this same scenario, we can create a new instance of the AbortController
object and pass fetch
a reference to the AbortController
instance’s signal
object.
Here’s what I mean:
const controller = new AbortController();
const signal = controller.signal;
fetch('https://api.github.com/users/nas5w', { signal })
.then((res) => res.json())
.then((data) => {
console.log(data);
});
So now, fetch
has a reference to the signal
object on our controller instance. We can abort our fetch by calling abort
on our controller:
const controller = new AbortController();
const signal = controller.signal;
fetch('https://api.github.com/users/nas5w', { signal })
.then((res) => res.json())
.then((data) => {
console.log(data);
});
controller.abort();
If we run this, we no longer log the returned data because we have immediately aborted our fetch
request!
Handling the Cancellation
You may have noticed in our last code snippet that our fetch
request isn’t gracefully aborted, we actually see an error in our console:
Uncaught (in promise) DOMException: The user aborted a request.
In order to handle this cancellation error, we simply need to catch
our error:
const controller = new AbortController();
const signal = controller.signal;
fetch('https://api.github.com/users/nas5w', { signal })
.then((res) => res.json())
.then((data) => {
console.log(data);
})
.catch((err) => {
console.log(err);
});
controller.abort();
Now if we run our code we see that we gracefully log our error:
DOMException: The user aborted a request.
So now we have successfully aborted our fetch request and caught the associated error.
A Note on Browser Compatibility
Most modern browsers have full support for AbortController
but (of course) if you have to support IE you’re out of luck! Be sure to check out the associated MDN docs for full compatibility info.
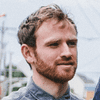
Nick Scialli is a senior UI engineer at Microsoft.