How to Abort Multiple Fetch Requests in JavaScript using AbortController
Nick Scialli
December 08, 2020
We might want to use AbortController to abort multiple fetch requests in JavaScript. How might we accomplish this?
A Quick Refresh: Aborting One Request
Let’s quickly refresh ourselves on how to abort one fetch
request using AbortController
. We first create a new instance of AbortController
. Then, we pass the instance’s signal
property in the second argument of the fetch
function call. Finally, calling abort()
on our instance will cancel the request and throw an error that we can catch
.
const controller = new AbortController();
const signal = controller.signal;
fetch('https://api.github.com/users/nas5w', { signal })
.then((res) => res.json())
.then((data) => {
console.log(data);
})
.catch((err) => {
console.log(err);
});
controller.abort();
Canceling Multiple Requests
One question we need to answer when we think about canceling multiple fetch
requests is whether we want to cancel them at the exact same time, or whether we might want to cancel them independently (or at least have that option). With one instance of AbortController
we can accomplish the former but not the latter.
Canceling two fetch requests simultaneous
If we want to cancel two fetch
requests simultaneously, it’s as easy as passing our same signal
parameter to the second fetch
call as well! Here’s what I mean:
const controller = new AbortController();
const signal = controller.signal;
// First fetch call
fetch('https://api.github.com/users/nas5w', { signal })
.then((res) => res.json())
.then((data) => {
console.log(data);
})
.catch((err) => {
console.log(err);
});
// Second fetch call
fetch('https://api.github.com/users/octocat', { signal })
.then((res) => res.json())
.then((data) => {
console.log(data);
})
.catch((err) => {
console.log(err);
});
controller.abort();
And we’ve canceled both requests!
Canceling the requests independently
While we could use the same AbortController
, and therefore the same AbortSignal
to cancel two requests simultaneously, we’ll need two different AbortController
instances if we want to cancel two requests independently.
const firstController = new AbortController();
const firstSignal = firstController.signal;
// First fetch call
fetch('https://api.github.com/users/nas5w', { signal: firstSignal })
.then((res) => res.json())
.then((data) => {
console.log(data);
})
.catch((err) => {
console.log(err);
});
const secondController = new AbortController();
const secondSignal = secondController.signal;
// Second fetch call
fetch('https://api.github.com/users/octocat', { signal: secondSignal })
.then((res) => res.json())
.then((data) => {
console.log(data);
})
.catch((err) => {
console.log(err);
});
// Abort the first request
firstController.abort();
// Abort the second request
secondController.abort();
And that’s all there is to it! You just use one instance AbortController
if you’re canceling the requests simultaneously, otherwise you create two separate instances.
Browser Compatibility
Most modern browsers have full support for AbortController
but (of course) if you have to support IE you’re out of luck! Be sure to check out the associated MDN docs for full compatibility info.
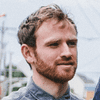
Nick Scialli is a senior UI engineer at Microsoft.