How to add script tags to your React app
Nick Scialli
March 14, 2023
If you have tried to add script tags to your React application, you may have noticed that it’s not as simple as adding <script src="..."></script>
to your JSX. In this post, we’ll cover a few different ways to get the task done.
If your script is static
If you have a script that should be added to your application no matter what, and that doesn’t need to be conditionally added or modified, the easiest way to add it is to put it directly to the index.html
file. Your index.html
file probably looks something like this:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<link rel="icon" type="image/svg+xml" href="/vite.svg" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>My React App</title>
</head>
<body>
<div id="root"></div>
<script type="module" src="/src/main.jsx"></script>
</body>
</html>
You already have a single script
tag that requires your main React javascript bundle into the application. If you need to require another script
, you can add it next to this existing script:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<link rel="icon" type="image/svg+xml" href="/vite.svg" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>My React App</title>
</head>
<body>
<div id="root"></div>
<script type="module" src="/src/main.jsx"></script>
<script src="path/to/some-other-script.js"></script>
</body>
</html>
And that’s a really quick solution, but only works if your script is static (and if you want it to load no matter what page someone lands on).
If your script is dynamic
Dynamic scripts are a bit trickier. It’s possible you only want to include a script when a user hits a certain component, or perhaps the script source is different depending on a few different factors.
The good news is we have a couple ways we can handle this situation!
Using an effect
In the component where you want to trigger the script load, you can use an effect that dynamically creates a script
element and adds it to the DOM. For example:
import { useEffect } from 'react';
function MyComponent() {
useEffect(() => {
const script = document.createElement('script');
script.setAttribute('src', 'path/to/some-script.js');
document.appendChild(script);
}, []);
return <>Some component content</>;
}
When the component first renders, this effect runs. We create a script element, set its src
attribute to the appropriate URL, and then append the script to the document. Once it’s appended to the document, the browser will fetch, parse, and execute the javascript!
Using React Helmet
There’s a very helpful npm package named react-helmet that can manage changes to the document head. Side note: this package was made by the NFL and the name “helmet” is so fitting!
Anyways, if we want to install react-helmet
in our application, we can run the following command in our terminal (assuming we’re using npm
… use the appropriate command for your package manager!).
npm install react-helmet
Then, inside the component, we can use the Helmet
component exported from react-helmet
:
import { Helmet } from 'react-helmet';
function MyComponent() {
return (
<>
<Helmet>
<script src="path/to/some-script.js"></script>
</Helmet>
Some component content
</>
);
}
This is a really neat approach because we can write our script
tag just like we would in HTML. Nice and declarative!
Conclusion
Adding a script
to your React project isn’t as straightforward as adding it directly to your JSX, but there are certainly a number of ways to accomplish the task. Happy coding!
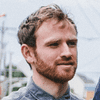
Nick Scialli is a senior UI engineer at Microsoft.