How to Add a Thousandths Place Comma (Every Three Digits) in JavaScript
Nick Scialli
December 29, 2020
Let’s do some basic number formatting for large numbers in JavaScript. If you’re here, you probably to be able to make the following conversions:
- 1000 -> 1,000
- 1000000 -> 1,000,000
- 100 -> 100
You’re in luck: there are a couple fairly simple ways to do this in JavaScript
toLocaleString
One simple option is the built-in toLocaleString
string method. It goes something like this:
(1000).toLocaleString('en-US');
// 1,000
(1000000).toLocaleString('en-US');
// 1,000,000
(100).toLocaleString('en-US');
// 1000
Regular Expressions
Another option is to use a Regular Expression. If our input is a number, we’ll want to make sure to covert it to a string before applying the regex.
function addCommas(num) {
return num.toString().replace(/(\d)(?=(\d\d\d)+(?!\d))/g, '$1,');
}
addCommas(1000);
// 1,000
addCommas(1000000);
// 1,000,000
addCommas(100);
// 1000
And that’s it! A couple fairly straightforward ways to add commas to your large numbers.
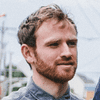
Nick Scialli is a senior UI engineer at Microsoft.