How to Use Pipe (the Pipeline Operator) in JavaScript
Nick Scialli
April 19, 2021
The pipeline operator is common in functional programming. There’s no built-in JavaScript pipeline operator (yet), but we can easily roll our own.
What is Piping?
Piping is taking the output of one function and sending it directly into another function. In pseudocode, this can be represented as:
output = input -> func1 -> func2 -> func3
In this case, the input
is piped into func1
, the output of of func1
is piped into func2
, and the output of func2
is piped into func3
.
In JavaScript, we might do this without piping by doing the following:
const output = func3(func2(func1(input)));
This is hard to read and unintuitive! While it’d be great if we could use the actual pipeline operator (|>
) in JavaScript, it’s not actually available to us… yet. It is coming, but more on that later!
Rolling Your Own
The good news is that we can roll our own pretty quickly while we’re waiting. In fact, it can be written as a handy one-liner!
const pipe = (...args) => args.reduce((acc, el) => el(acc));
const output = pipe(input, func1, func2, func3);
…and that’s it! But how does this work? Well, first, we use rest parameters (...args
) to allow for any number of parameters to be passed into our pipe
function and those parameters will be in the array args
.
Next, we use the Array.reduce
method to iterate though the array of args
. As we reduce
, the accumulator becomes the result of passing the previous accumulator to the current element!
A Practical Example
Let’s look at a practical example: converting a blog post title into a slug. We’re going to start our with a string that’s title-cased (e.g., "10 Weird Facts About Dogs"
) and convert it into a slug (e.g., "10-weird-facts-about-dogs"
). To do this, we’ll wan’t to pipe through a couple functions:
title |> lowercase |> addHyphens
In JavaScript, we can do this with our custom pipe
function:
const pipe = (...args) => args.reduce((acc, el) => el(acc));
const title = '10 Weird Facts About Dogs';
const toLowerCase = (str) => str.toLowerCase();
const addHyphens = (str) => str.replace(/\s/g, '-');
const slug = pipe(title, toLowerCase, addHyphens);
console.log(slug);
// 10-weird-facts-about-dogs
The Actual Pipeline Operator
At the time of writing this post (April 19, 2021), the actual pipeline operator (|>
) is in draft/stage 1 of TC39 consideration. If you’re really itching to use it, There is a Pipeline Proposal Babel Plugin you can experiment with!
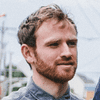
Nick Scialli is a senior UI engineer at Microsoft.