Short-Circuit Evaluation in JavaScript
Nick Scialli
September 07, 2019
Introduction
Traditionally, we’re used to using if-else
statements in JavaScript to perform conditionally variable assignment and handle control flow. In this post, we’ll explore using short-circuit evaluation to accomplish the same tasks.
What is Short-Circuit Evaluation?
The syntax of short-circuit evaluation is already somewhat familiar; it uses the ||
or &&
logical operators you would normally see in if-else
statements… but used outside of if-else
. The ||
operator can be used to return the first truthy value in the expression while the &&
operator can be used to prevent execution of subsequent code.
The
||
operator can be used to return the first truthy value in the expression while the&&
operator can be used to prevent execution of subsequent code.
Some Examples
Using the || operator
Here’s an example of an if-else
statement converted to a short-circuit evaluation. In this example, we want to get a display name for our user. If the user
object has a name
property, we will use that as a display name. Otherwise, we will just call our user “Guest.”
const user = {};
let displayName;
if (user.name) {
displayName = user.name;
} else {
displayName = 'Guest';
}
console.log(displayName); // "Guest"
This can be simplified greatly using short-circuit evaluation:
const user = {};
const displayName = user.name || 'Guest';
console.log(displayName); // "Guest"
Since user.name
is undefined
, it’s falsey. “Guest” is therefore returned from the short-circuit evaluation! Conversely, if user.name
existed and was not falsey, it would be returned:
const user = { name: 'Daffodil' };
const displayName = user.name || 'Guest';
console.log(displayName); // "Daffodil"
What’s really neat is we can chain these conditions. Let’s say we also potentially have a user’s nickname. Our if-else
conditional might start getting pretty ugly:
const user = { name: 'Joe Smith' };
let displayName;
if (user.nickname) {
displayName = user.nickname;
} else if (user.name) {
displayName = user.name;
} else {
displayName = 'Guest';
}
console.log(displayName); // "Joe Smith"
Using short-circuit evalution, this all becomes much simpler:
const user = { name: 'Joe Smith' };
const displayName = user.nickname || user.name || 'Guest';
console.log(displayName); // "Joe Smith"
Much nicer!
Using the && operator
The &&
short-circuit works a bit differently. With the &&
operator, we can make sure subsequent expressions are only run if previous expressions are truthy. What does this mean exactly? Let’s look at another example! In this example, we want to show an error in our console if a server response is not successful. First, we can do this using and if
statement.
const response = { success: false };
if (!response.success) {
console.error('Oh no!');
}
Using a short-circuit evalution, the same could be accomplished as follows:
const response = { success: false };
!response.success && console.error('Oh no!');
If response.success
is true, then !response.success
is false. Therefore, the entire expression will evaluate to false
and there is no need for the interpretor to look at anything to the right of the &&
.
Potential Gotcha: Legitimate Falsey Values
Let’s say your app may or may not know the number of children a user has. You might think of using the following short-circuit evaluation:
const numberOfChildrenDisplay = user.numberOfChildren || 'Unknown';
The problem here is that 0
is a legitimate value of a known number of children, but 0
is falsey! So the following behavior would be incorrect:
const user = { numberOfChildren: 0 };
const numberOfChildrenDisplay = user.numberOfChildren || 'Unknown';
console.log(numberOfChildrenDisplay); // "Unknown"
Conclusion
Short-circuilt evaluations can be great shorthand for variable assignment and control flow, just make sure you consider the validity of falsey values when you use them!
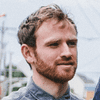
Nick Scialli is a senior UI engineer at Microsoft.