The JavaScript Set Object
Nick Scialli
February 19, 2020
Set
is one of my favorite built-in object types in JavaScript. Today I’ll introduce the Set
object and discuss some of its use cases.
The Set Object
The Set
object is a collection of values in which you can store unique primitive values or object references. Uniqueness is key here—no primitive value or object reference will can added multiple times.
How to Use Set
To use set, you create a new instance of it.
const mySet = new Set();
We now have an empty set. We can add the number 1
to this set by using the add
method.
mySet.add(1);
How do we know that we’ve added 1
? We can use the has
method to check.
console.log(mySet.has(1));
// true
Let’s add an object reference now and then check that we have that object in our Set
.
const obj = { name: 'Daffodil' };
mySet.add(obj);
console.log(mySet.has(obj));
// true
Remember that object references are compared, not the object keys themselves. In other words:
console.log(mySet.has({ name: 'Daffodil' }));
// false
We can see how many elements are in the Set
by using the size
property.
console.log(mySet.size);
// 2
Next up, let’s remove a value using the delete
method.
mySet.delete(1);
console.log(mySet.has(1));
// false
Finally, we’ll clear out the Set
using the clear
method.
mySet.clear();
console.log(mySet.size);
// 0
Iterating Over a Set
The easiest way to iterate over a Set
is to use the forEach
method.
new Set([1, 2, 3]).forEach((el) => {
console.log(el * 2);
});
// 2 4 6
Set
objects also have entries
, keys
, and values
methods, which each returns Iterators. Those are a bit outside the scope of this tutorial!
Using Sets in the Wild
I find the Set
object to be really great for keeping track of a binary state associated with an object. A great example is an accordion menu: each item in the menu will either be open or closed. We can create a Set
called isOpen
that tracks the open status of an accordion item and a toggle
function that toggles the open status:
const isOpen = new Set();
function toggle(menuItem) {
if (isOpen.has(menuItem)) {
isOpen.delete(menuItem);
} else {
isOpen.add(menuItem);
}
}
A Note of Efficiency
You might be thinking that the Set
object seems awfully similar to arrays. There is, however, a big difference that may have performance ramifications in your application. The Set
object is required to be implemented using hash tables (or methods with hash table-like efficiency) [1].
When you store something in an array, you might have to traverse the entire array to find the item. With a Set
, however, the lookup is instantaneous. Practically speaking, the performance will be negligable for most cases, but good to remember if you find yourself having to track large numbers of items!
Conclusion
I hope this helped you understand the Set
object and you now have a new tool in your JavaScript toolbelt!
References
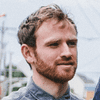
Nick Scialli is a senior UI engineer at Microsoft.