Why is My Recursive Function Returning Undefined in JavaScript?
Nick Scialli
November 13, 2020
One common frustration when getting the hang of recursion is not understanding why your recursive function is returning undefined.
Often, this is because you’re not return
ing the next execution of your recursive function. I’ll show you what I mean.
Let’s say we’re writing a factorial function. Factorial is a mathematical operator where a number is multiplied by each lower number. For example, 4 factoral (written as 4!) is equivalent to 4 * 3 * 2 * 1 = 24.
We might write a recursive factorial function like this:
function factorial(num, result = 1) {
if (num === 1) {
return result;
}
factorial(num - 1, num * result);
}
console.log(factorial(4));
// undefined
But this should be 24
! Why is it undefined
? Well the answer is easier than we think: we need to return
the next execution of the factorial
function each time or else we’ll never get to the ultimate result!
function factorial(num, result = 1) {
if (num === 1) {
return result;
}
return factorial(num - 1, num * result);
}
console.log(factorial(4));
// 24
And that’s really it! The “secret” is to just make sure that, whatever path you go down in your function, make sure you’re return
ing something.
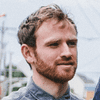
Nick Scialli is a senior UI engineer at Microsoft.