Why We're Looking Forward to Optional Chaining
Nick Scialli
July 28, 2019
Introduction
Recently, we found out that the TC39 committee, which is responsible for the ECMAScript language standard, advanced Optional Chaining to Stage 3 of consideration for inclusion in the standard. This is the second-to-last stage of consideration, so a lot of people got excited about this. Today, I’ll explore Optional Chaining and the problem it solves.
First, the Problem
In JavaScript, accessing properties in objects can be dangerous if we aren’t positive about the object’s structure. Let’s say we have a user
object that may or may not have membership information. If we just assume our user has membership information, we can get into trouble.
const user = { name: 'Jen' };
const membershipType = user.membership.type;
// TypeError: Cannot read property 'type' of undefined
There are a couple ways to protect against this happening. We can explicitly check to see if the membership
property exists:
let membershipType = undefined;
if (user.membership) {
membershipType = user.membership.type;
}
Or we can use a short circuit operator that tests whether user.membership
exists:
const membershipType = user.membership && user.membership.type;
I prefer the latter approach, but imagine how this looks when you need to drill down several levels:
const membershipTypeName =
user.membership && user.membership.type && user.membership.type.name;
This starts getting pretty out-of-hand.
So, What is Optional Chaining?
Optional Chaining is essentially shorthand for the latter approach. Let’s rewrite the last snippet using the proposed Optional Chaining approach:
const membershipTypeName = user?.membership?.type?.name;
In this case, we’re letting JavaScript know that we’re not quite sure whether there’s a membership
property on user
, whether there’s a type
property on membership
, or whether there’s a name
property on type
. If any of these checks is untrue, our membershipTypeName
variable will end up undefined
rather than throwing an error.
Conclusion
There’s quite a bit more to the Optional Chaining proposal, but this is the general idea!
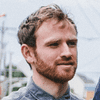
Nick Scialli is a senior UI engineer at Microsoft.