How to capitalize the first letter of a string in JavaScript
Nick Scialli
March 20, 2023
If you’ve tried to capitalize the first letter of a string in JavaScript, you may have found it to not be so trivial! Read on to learn how to accomplish this task.
Strings are immutable
It’s important to understand that strings, like other primitives in JavaScript, are immutable. What does this mean? Well it means that you can just reassign a letter in a string. For example, the following won’t work:
// X - this won't work!
const name = 'frank';
name[0] = 'F';
We need to assemble the pieces
Since strings are immutable, we’ll need to approach this problem by creating a new string out of two pieces:
- A capitalized version of the first letter
- The remainder of the string with the first letter removed
Here’s a solution that will work:
function capitalizeFirstLetter(str) {
return str[0].toUpperCase() + str.slice(1);
}
const name = 'frank';
const newName = capitalizeFirstLetter(name);
console.log(newName);
// "Frank"
How does this work?
str[0]
represents the letter of the string at index 0. In this case, that’s "f"
. We do "f".toUpperCase()
, which converts "f"
to "F"
. Part one done!
str.slice(1)
is a bit trickier. What the slice
method does is returns everything in the string from the first index provided to the second index provided. In this case, we don’t provide a second index, so slice
goes until the end of the word. This is how we end up with "rank"
.
Finally, "F" + "rank"
is a string concatenation, leaving us with "Frank"
.
How to capitalize the first letter of every word in a sentence?
Since we already know how to capitalize the first letter of a word, we can use this existing function to accomplish a more complicated task: capitalizing the first letter of each word in a sentence.
We’ll do this with the following algorithm:
- Break the sentence into words
- Capitalize the first letter of each of those words
- Reassemble the sentence
Let’s see it in action!
function capitalizeFirstLetter(str) {
return str[0].toUpperCase() + str.slice(1);
}
function capitalizeWords(sentence) {
const words = sentence.split(' ');
const capitalizedWords = words.map((word) => capitalizeFirstLetter(word));
const reassembed = capitalizedWords.join(' ');
return reassembed;
}
const sentence = 'steph went to the market';
console.log(capitalizeWords(sentence));
// "Steph Went To The Market"
And there you have it, you can now capitalize the first letter of a word (or all of them in a sentence)!
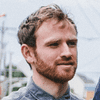
Nick Scialli is a senior UI engineer at Microsoft.