How to sort an array of objects by multiple properties in JavaScript
Nick Scialli
March 20, 2023
The Array.sort
method can be challenging to grok, especially when you have to sort multiple properties. In this post, we’ll go through the mechanics and have you sorting like a pro!
How the sort method works generally
The sort
method is called directly on an array and it mutates the array. So for example, the following code demonstrates an array being sorted in-place:
const arr = ['d', 'b', 'a', 'c'];
arr.sort();
console.log(arr);
// ["a", "b", "c", "d"]
For this basic use case, calling sort
without any arguments is sufficient. But in many cases it won’t suffice. When you need more complex comparisons between elements being sorted, you need to provide a compare function.
The compare function takes two arguments: the first item being compared and the second item being compared. The compare function should return a number.
- If the returned number is less than zero, the first item being compared is sorted forst
- If the returned number is greater than zero, this second item being compared is sorted first
- If the returned number is zero, the original ordering is preserved
A fairly common use case is numerical sorting. For example, we can write the following:
const arr = [2, 3, 1, 4, 3];
arr.sort((a, b) => a - b);
console.log(arr);
// [1, 2, 3, 3, 4]
Sorting objects by a property
Let’s create a list of people with names and ages. We want to sort these people by their ages in ascending order. This actually ends up being fairly similar to our last example except this time we sort each element of the array using a property:
const arr = [
{ name: 'Mike', age: 20 },
{ name: 'Peter', age: 30 },
{ name: 'Doris', age: 18 },
];
arr.sort((a, b) => a.age - b.age);
console.log(arr);
/*
[
{ name: 'Doris', age: 18 },
{ name: 'Mike', age: 20 },
{ name: 'Peter', age: 30 },
]
*/
Sorting objects by multiple properties
Finally, we have our goal case: sorting objects by multiple properties. Let’s use our people example again, except this time we’ll have two people with an age of 20. When that happens, we don’t want the order to be random, we want the first names to be alphabetical! So how can we do this multiple sorting?
It’s a matter of adjusting our comparison function. We’ll want to make sure the comparison function first sorts by age. If the age is the same, we’ll compare the name fields.
const arr = [
{ name: 'Mike', age: 20 },
{ name: 'Peter', age: 30 },
{ name: 'Doris', age: 18 },
{ name: 'Edgar', age: 30 },
];
arr.sort((a, b) => {
// Only sort on age if not identical
if (a.age < b.age) return -1;
if (a.age > b.age) return 1;
// Sort on name
if (a.name < b.name) return -1;
if (a.name > b.name) return 1;
// Both idential, return 0
return 0;
});
console.log(arr);
/*
[
{ name: 'Doris', age: 18 },
{ name: 'Mike', age: 20 },
{ name: 'Edgar', age: 30 },
{ name: 'Peter', age: 30 },
]
*/
And there we have it! The people are sorted by age. In this case, since Edgar and Peter have the same age, Edgar is first because his name comes earlier in the alphabet.
Conclusion
The sort
method in javascript can be hard to grok, but once you get the hang of how the compare function works you can do some pretty powerful things with it.
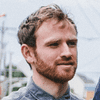
Nick Scialli is a senior UI engineer at Microsoft.