How to use onChange in Solid.js
Nick Scialli
February 22, 2022
If you’re used to React, you might be wondering why the Solid onChange handler doesn’t work how you’d expect.
The problem
You may be writing a Solid component with the following code:
function App() {
const [text, setText] = createSignal('');
return (
<>
<input
onChange={(e) => {
setText(e.target.value);
}}
/>
<div>
<strong>Your text is:</strong> {text}
</div>
</>
);
}
But you’ll quickly notice things aren’t working as you might expect:
The text
isn’t reflected in the div
like we would hope until after we tab out of the input. This is actually intended behavior and more in line with native behavior.
From the Solid.js docs:
Note that onChange and onInput work according to their native behavior. onInput will fire immediately after the value has changed; for input fields, onChange will only fire after the field loses focus.
The solution
As described above, you’re looking for the onInput
handler, which more closely mirrors native oninput behavior:
function App() {
const [text, setText] = createSignal('');
return (
<>
<input
onInput={(e) => {
setText(e.target.value);
}}
/>
<div>
<strong>Your text is:</strong> {text}
</div>
</>
);
}
Now if we run our app, we see the text
updating immediately:
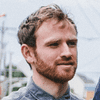
Nick Scialli is a senior UI engineer at Microsoft.